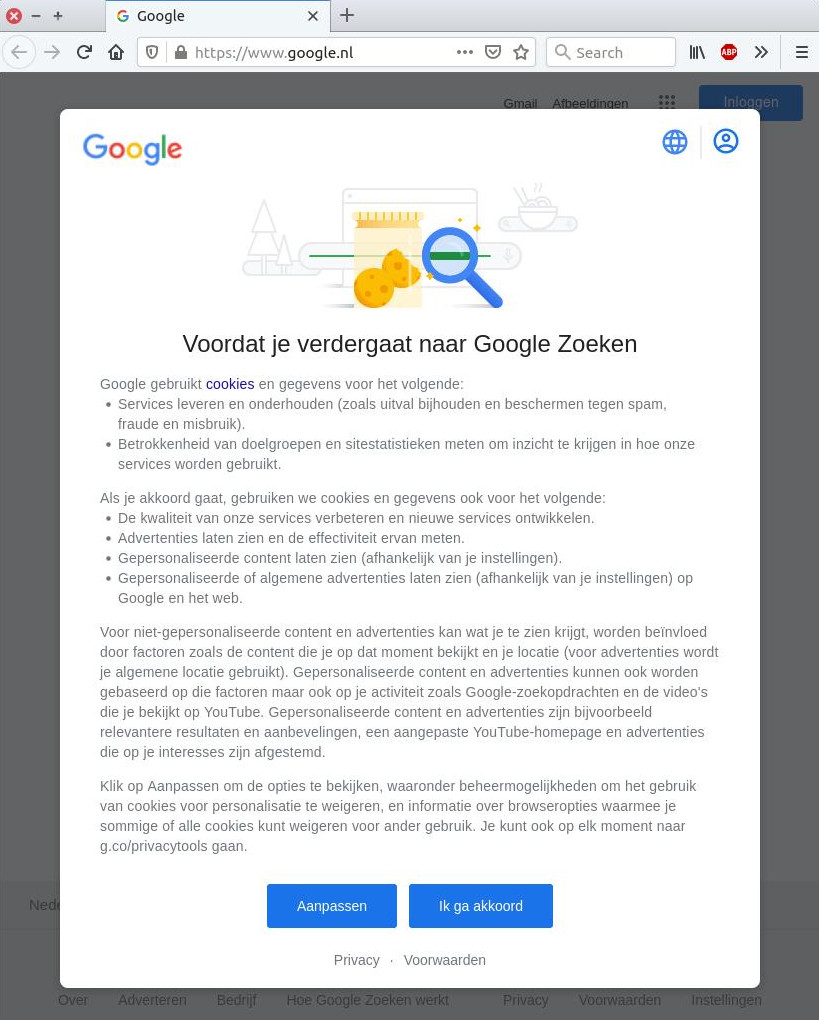
If you automatically delete cookies whenever you close your browser, you will have to answer the cookie consent message every time you use Google search.
With a single click you can give away your privacy. Not willing to do that? Then you have to manually go through a list of options with no default values, a tedious process clearly designed to discourage you.
The Tampermonkey script below automatically declines all personalization options. This script addresses the Dutch and English language dialogs, but can be easily adapted to other languages.
Google consent Tampermonkey script
// ==UserScript==
// @name Google consent
// @namespace http://tampermonkey.net/
// @version 1.0
// @description Auto fill cookie consent
// @run-at document-idle
// @match https://www.google.nl/*
// @match https://www.google.com/*
// @match https://consent.google.nl/*
// @match https://consent.google.com/*
// @icon https://www.google.com/s2/favicons?domain=google.com
// @grant none
// ==/UserScript==
function clickEvent() {
return new MouseEvent('click', {
view: window,
bubbles: true,
cancelable: true
});
}
(function() {
'use strict';
setTimeout(function() {
for( let el of Array.from(document.querySelectorAll('div')).filter(el => el.textContent === 'Aanpassen' || el.textContent.startsWith('Customi')) ) {
el.dispatchEvent(clickEvent());
}
var c = Array.from(document.querySelectorAll('label')).filter(el => el.textContent === 'Uit' || el.textContent === 'Off');
if( c.length == 0 ) {
c = Array.from(document.querySelectorAll('span')).filter(el => el.textContent === 'Uit' || el.textContent === 'Off');
}
if( c.length > 0 ) {
for( let el of c ) {
el.dispatchEvent(clickEvent());
}
setTimeout(function() {
for( let el of Array.from(document.querySelectorAll('input')).filter(el => el.value === 'Bevestigen' || el.value === 'Confirm') ) {
el.dispatchEvent(clickEvent());
}
for( let el of Array.from(document.querySelectorAll('span')).filter(el => el.textContent === 'Bevestigen' || el.textContent === 'Confirm') ) {
el.dispatchEvent(clickEvent());
}
}, 50);
}
}, 50);
})();